티스토리 뷰
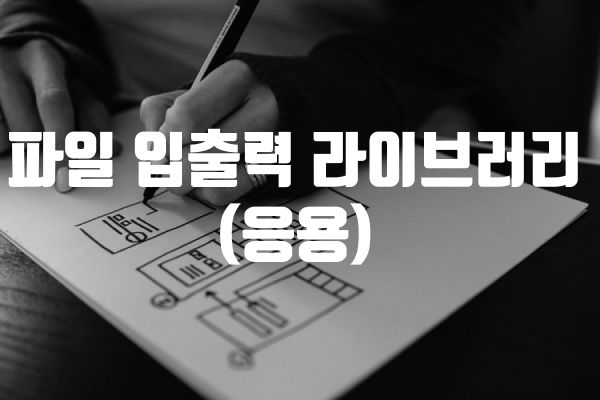
파일 입출력 라이브러리(응용)
# 텍스트 파일 입출력
1. fscanf
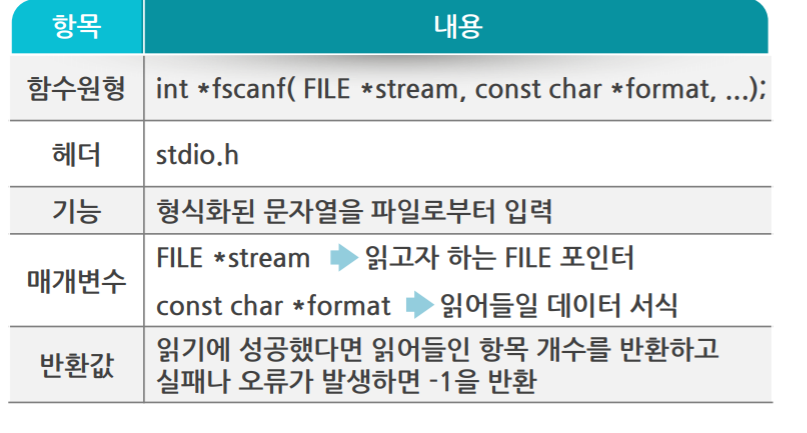
%o : 8진 정수 형식으로 입력
%d : 10진 정수 형식으로 입력
%ld : long형 10진 정수 형식으로 입력
%x : 16진 정수 형식으로 입력
%f : float형 형식으로 입력
%lf : double형 형식으로 입력
%c : 문자 형식으로 입력
%s : 문자열 형식으로 입력
2. rewind

3. fprintf

형식 문자열의 구조
%[*] [width] [{h ¦ 1 ¦ L}]type
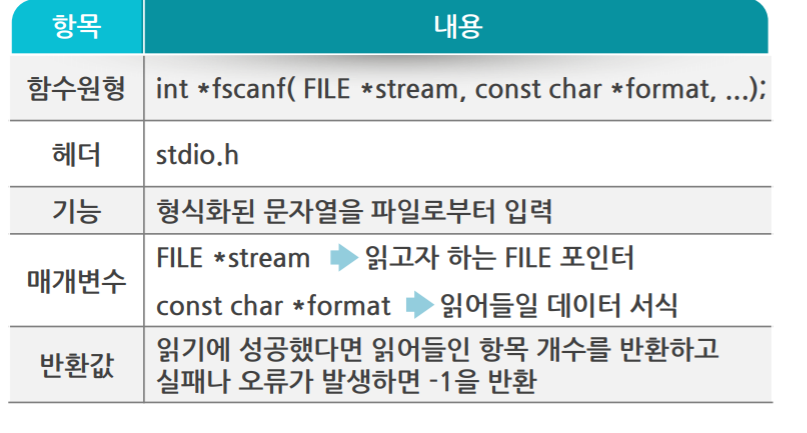
%d : 10진 정수 형식으로 입력
%ld : long형 10진 정수 형식으로 입력
%x : 16진 정수 형식으로 입력
%f : float형 형식으로 입력
%lf : double형 형식으로 입력
%c : 문자 형식으로 입력
%s : 문자열 형식으로 입력
#include
int main( void)
{
FILE *fp;
char buf[1024];
int num;
float real;
if ( fp = fopen( “data.txt”, “r”)) {
while( 0 < fscanf( fp, “%d %f %s”, &num, &real, buf))
printf( “%d %f %s\n”, num, real, buf);
fclose( fp);
}
return 0;
}
//결과값
123 456.789001
hello
1 123.456001 good
100 456.455994
morning

#include
#include
int main () {
char str1[10], str2[10], str3[10];
int year;
FILE * fp;
fp = fopen (“file.txt”, “w+”);
fputs("We are in 2020", fp);
rewind(fp);
fscanf(fp, “%s %s %s %d”, str1, str2, str3,&year);
printf(“Read String1 |%s|\n”,str1 );
printf(“Read String2 |%s|\n”,str2 );
printf(“Read String3 |%s|\n”,str3 );
printf(“Read Integer |%d|\n”,year );
fclose(fp);
return(0);
}
// 결과값
Read String1 |We|
Read String2 |are|
Read String3 |in|
Read Integer |2020|

#include
int main ()
{
FILE * pFile;
int n;
char name [100];
pFile = fopen (“myfile.txt”, “w”);
for (n=0 ; n < 3 ; n++){
puts (“please, enter a name: ”);
gets (name);
fprintf (pFile, “Name %d [%-10.10s]\n”,n+1,name);
}
fclose (pFile);
return 0;
}
// 결과값
Name 1 [kim ]
Name 2 [choi ]
Name 3 [park ]
# 이진 파일 입출력
1. 파일 열기
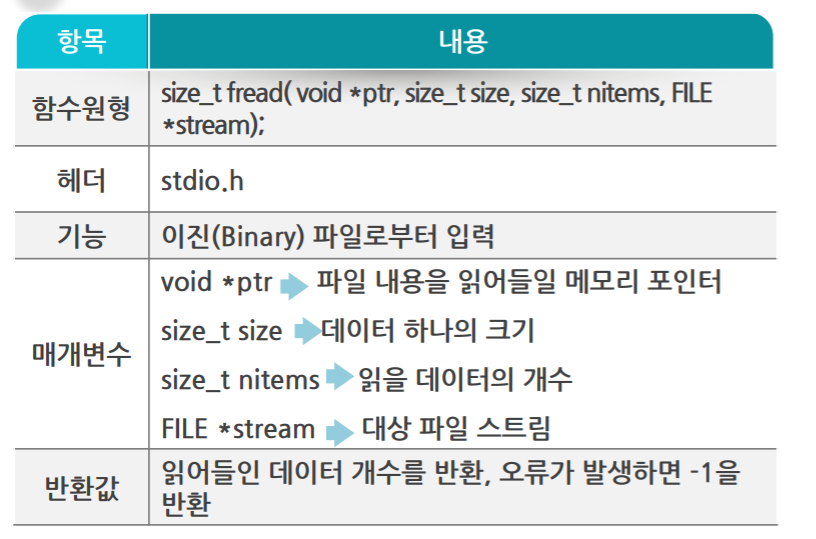
2. fwrite

3. fseek

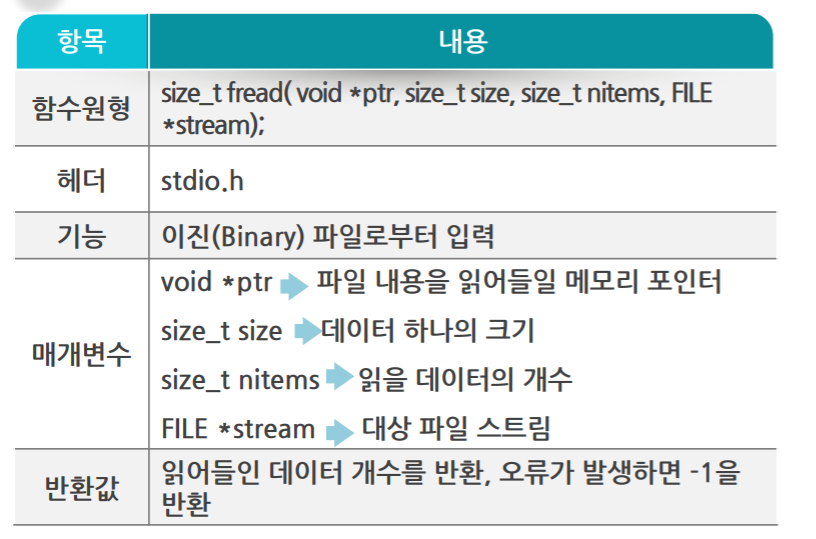
#include
#include
int main () {
FILE * pFile;
char buffer[500];
size_t result;
pFile = fopen ( “myfile.bin” , “rb” );
if (pFile==NULL) {
fputs (“File error”,stderr); exit (1);
}
fread (buffer,1,lSize,pFile);
………
fclose (pFile);
return 0;
}

#include
enum { SIZE = 5 };
int main(void)
{
double a[SIZE] = {1.,2.,3.,4.,5.};
FILE *fp = fopen("test.bin", "wb"); // must use binarymode
fwrite(a, 8, SIZE, fp); // writes an array of doubles
fclose(fp);
double b[SIZE];
fp= fopen("test.bin","rb");
size_tret_code= fread(b, 8, SIZE, fp); // reads an array of doubles
if(ret_code== SIZE) {
puts("Array read successfully, contents: ");
for(intn = 0; n < SIZE; ++n) printf("%f ",b[n]);
putchar('\n');
} else { // error handling
if (feof(fp))
printf("Error reading test.bin: unexpected end of file\n");
else if (ferror(fp)) {
perror("Error reading test.bin");
}
fclose(fp);
}
intmain( void)
{
FILE *fp_sour; // 파일원본을위한파일스트림포인터
FILE *fp_dest; // 복사대상을위한파일스트림포인터
char buff[1024]; // 파일내용을읽기/쓰기를위한버퍼
size_t n_size; // 읽거나쓰기를위한데이터의개수
fp_sour = fopen( "./main.c" , "r");
fp_dest = fopen( "./main.bck", "w");
while( 0 < (n_size= fread( buff, 1, 1024, fp_sour))) {
fwrite( buff, 1, n_size, fp_dest);
}
fclose( fp_sour);
fclose( fp_dest);
return 0;
}

#include
int main ()
{
FILE * pFile;
pFile = fopen ( “example.txt” , “wb” );
fputs ( "This is an apple." , pFile );
fseek ( pFile , 9 , SEEK_SET );
fputs ( " sam" , pFile );
fclose ( pFile );
return 0;
}
// 결과값
This is a sample.
'JAVA기반스마트웹개발2021 > 프로그래밍언어 활용' 카테고리의 다른 글
시간 관련 라이브러리 (0) | 2021.08.09 |
---|---|
도서관리 시스템 고도화(파일 처리) (0) | 2021.08.08 |
파일 입출력 라이브러리(기초) (0) | 2021.08.08 |
도서관리 시스템 고도화(동적메모리) (0) | 2021.08.08 |
함수 포인터 (0) | 2021.08.08 |